Concept of Array in Java
An array is a collection of similar data types. Array is a container object that hold values of homogenous type. It is also known as static data structure because size of an array must be specified at the time of its declaration.
An array can be either primitive or reference type. It gets memory in heap area. Index of array starts from zero to size-1.
Features of Array
- It is always indexed. Index begins from 0.
- It is a collection of similar data types.
- It occupies a contiguous memory location.
Array Declaration
Syntax :
datatype[] identifier;
or
datatype identifier[];
Both are valid syntax for array declaration. But the former is more readable.
Example :
int[ ] arr;
char[ ] arr;
short[ ] arr;
long[ ] arr;
int[ ][ ] arr; // two dimensional array.
Initialization of Array
new
operator is used to initialize an array.
Example :
int[] arr = new int[10]; //this creates an empty array named arr of integer type whose size is 10.
or
int[] arr = {10,20,30,40,50}; //this creates an array named arr whose elements are given.
Accessing array element
As mention ealier array index starts from 0. To access nth element of an array. Syntax
arrayname[n-1];
Example : To access 4th element of a given array
int[ ] arr = {10,20,30,40};
System.out.println("Element at 4th place" + arr[3]);
The above code will print the 4th element of array arr
on console.
Note: To find the length of an array, we can use the following syntax:
array_name.length
. There are no braces infront of length. Its not length().foreach or enhanced for loop
J2SE 5 introduces special type of for loop called foreach loop to access elements of array. Using foreach loop you can access complete array sequentially without using index of array. Let us see an example of foreach loop.
class Test
{
public static void main(String[] args)
{
int[] arr = {10, 20, 30, 40};
for(int x : arr)
{
System.out.println(x);
}
}
}
10
20
30
40
Multi-Dimensional Array
A multi-dimensional array is very much similar to a single dimensional array. It can have multiple rows and multiple columns unlike single dimensional array, which can have only one full row or one full column.
Array Declaration
Syntax:datatype[ ][ ] identifier;
or
datatype identifier[ ][ ];
Initialization of Array
new operator is used to initialize an array.
Example:int[ ][ ] arr = new int[10][10]; //10 by 10 is the size of array.
or
int[ ][ ] arr = {{1,2,3,4,5},{6,7,8,9,10},{11,12,13,14,15}};
// 3 by 5 is the size of the array.
Accessing array element
For both, row and column, the index begins from 0.
Syntax:array_name[m-1][n-1]
Example:int arr[ ][ ] = {{1,2,3,4,5},{6,7,8,9,10},{11,12,13,14,15}};
System.out.println("Element at (2,3) place" + arr[1][2]);
Jagged Array
Jagged means to have an uneven edge or surface. In java, a jagged array means to have a multi-dimensional array with uneven size of rows in it.
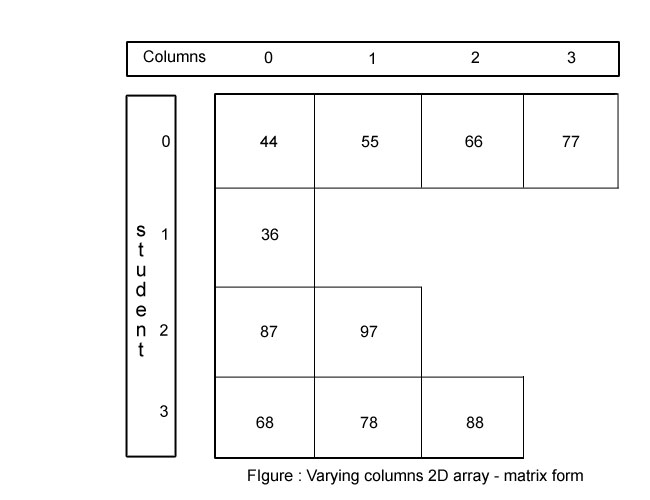
Initialization of Jagged Array
new operator is used to initialize an array.
Example:int[ ][ ] arr = new int[3][ ]; //there will be 10 arrays whose size is variable
arr[0] = new int[3];
arr[1] = new int[4];
arr[2] = new int[5];
0 comments:
Post a Comment